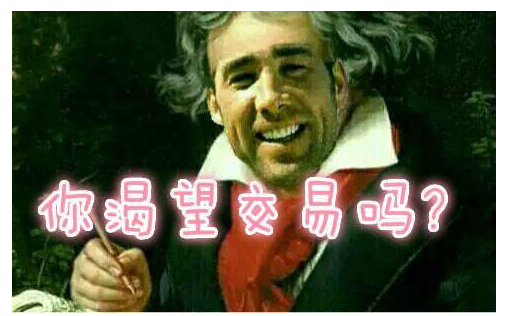
也可以来我的博客看哦崔斯特的博客专栏
1、使用Pygame
import pygame #导入Pygame
from pygame import * #导入Pygame中的所有常量
pygame.init() #初始化Pygame
screen = pygame.display.set_mode((600,500)) #创建窗口
myfont = pygame.font.Font(None,60) #创建字体对象
white = 255,255,255
blue = 0,0,255
textImage = myfont.render("hello Pygame",True,white)
screen.fill(blue)
screen.blit(textImage,(100,100))
pygame.display.update()
首先在内存中创建文本平面,然后再将文本当作一个图像来绘制。
运行程序,出现如下窗口,发现马上就关闭了,需要一个延迟。
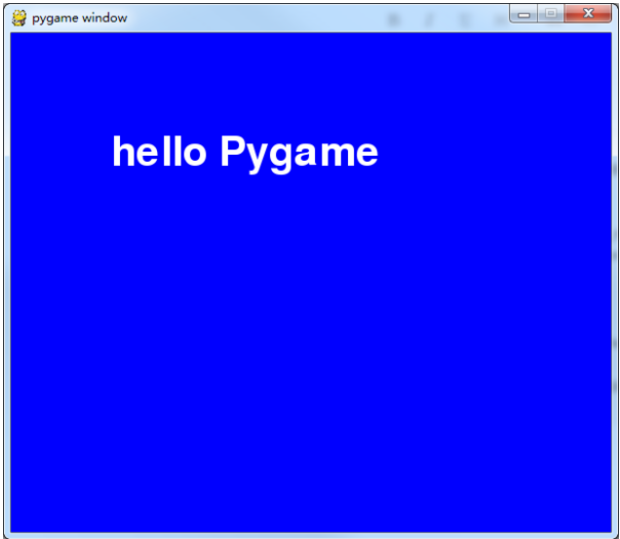
2、循环
import pygame,sys #导入Pygame
from pygame import * #导入Pygame中的所有常量
white = 255,255,255
blue = 0,0,255
pygame.init() #初始化Pygame
screen = pygame.display.set_mode((600,500)) #创建窗口
myfont = pygame.font.Font(None,60) #创建字体对象
textImage = myfont.render("hello Pygame",True,white)
while True: #加入循环
for event in pygame.event.get():
if event.type in (QUIT,KEYDOWN):
sys.exit()
screen.fill(blue)
screen.blit(textImage,(100,100))
pygame.display.update()
加入while循环,只要while条件为真,就会一直执行下去。
现在运行看看,还会自动关闭吗?
3、绘制圆
主要是使用 pygame.draw.circle()
import pygame,sys #导入Pygame
from pygame import * #导入Pygame中的所有常量
pygame.init() #初始化Pygame
screen = pygame.display.set_mode((600,500)) #创建窗口
pygame.display.set_caption("画圆")
while True:
for event in pygame.event.get():
if event.type in (QUIT,KEYDOWN):
sys.exit()
screen.fill((0,0,200))
#画圆
color = 255,255,0
position = 300,250
radius = 100
width = 10
pygame.draw.circle(screen,color,position,radius,width)
pygame.display.update()
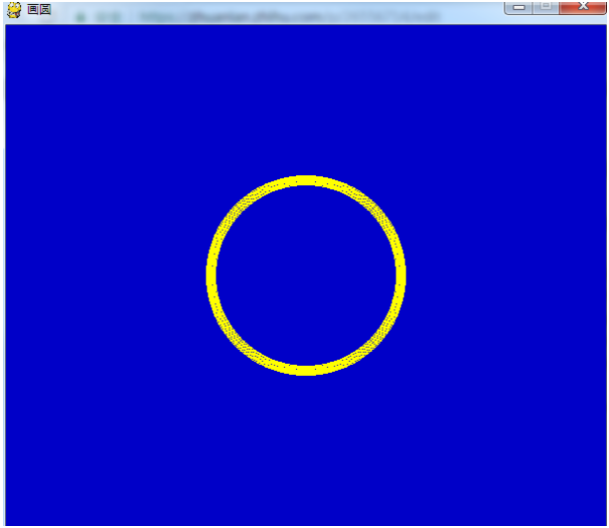
4、绘制矩形
通过多个参数来调用pygame.draw.rect()函数,在while循环之外的记录矩形的位置,并且创建一对速度变量。
import pygame,sys #导入Pygame
from pygame import * #导入Pygame中的所有常量
pygame.init() #初始化Pygame
screen = pygame.display.set_mode((600,500)) #创建窗口
pygame.display.set_caption("画矩形")
pos_x = 300
pos_y = 250
vel_x = 2
vel_y = 1
while True:
for event in pygame.event.get():
if event.type in (QUIT,KEYDOWN):
sys.exit()
screen.fill((0,0,200))
#移动矩形
pos_x += vel_x
pos_y += vel_y
#让矩形在屏幕中
if pos_x > 500 or pos_x < 0:
vel_x = -vel_x
if pos_y > 400 or pos_y < 0:
vel_y = -vel_y
#画矩形
color = 255,255,0
width = 0
pos = pos_x,pos_y,100,100
pygame.draw.rect(screen,color,pos,width)
pygame.display.update()
运行程序后,发现有一个矩形在窗口中跳来跳去,好像还有某种规律。。。
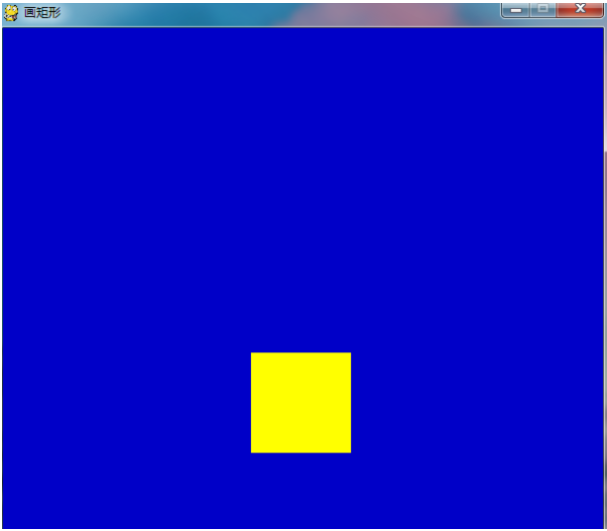
5、绘制线条
使用pygame.draw.line()函数来绘制直线,必须提供线条起点和终点
import pygame,sys #导入Pygame
from pygame import * #导入Pygame中的所有常量
pygame.init() #初始化Pygame
screen = pygame.display.set_mode((600,500)) #创建窗口
pygame.display.set_caption("画直线")
while True:
for event in pygame.event.get():
if event.type in (QUIT,KEYDOWN):
sys.exit()
screen.fill((0,80,0))
#画直线
color = 100,255,200
width = 8
pygame.draw.line(screen,color,(100,100),(500,400),width)
pygame.display.update()
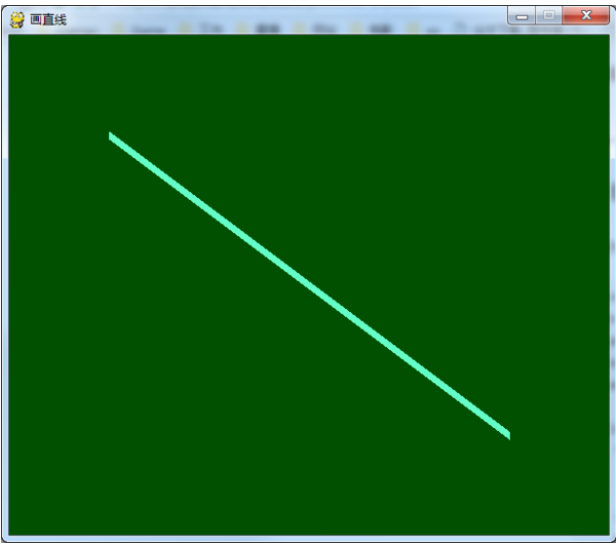
6、绘制弧线
弧线是圆的一部分,使用pygame.draw.arc()函数来绘制
要将角度转换弧度,使用math.radians()函数
import math,pygame,sys
from pygame.locals import *
pygame.init()
screen = pygame.display.set_mode((600,500))
pygame.display.set_caption("画弧线")
while True:
for event in pygame.event.get():
if event.type in (QUIT,KEYDOWN):
sys.exit()
screen.fill((0,0,200))
#画弧线
color = 255,0,255
position = 200,150,200,200
start_angle = math.radians(0)
end_angle = math.radians(180)
width = 8
pygame.draw.arc(screen,color,position,start_angle,end_angle,width)
pygame.display.update()
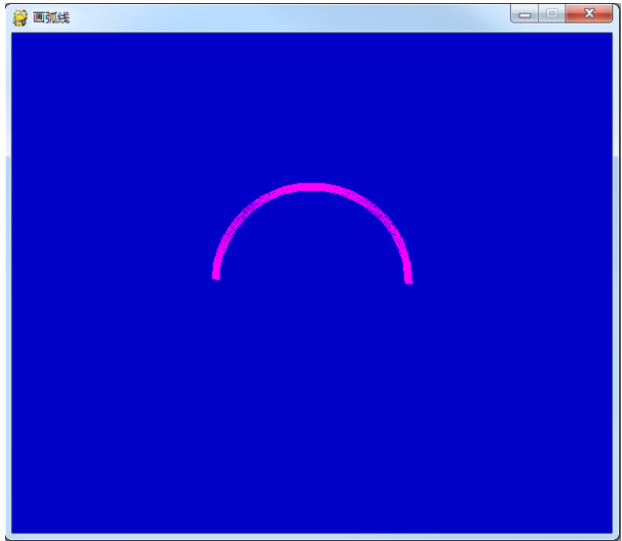
好啦,第一期的PY交易就结束了,小伙伴有什么想说的呢?评论中见
推荐阅读:http://www.pygame.org/news
本文参考Jonathan S.Harbour创作的《More Python Programming for the Absolute Beginner》More Python Programming for the Absolute Beginner
======================================================================为什么会玩Pygame呢?其实只是我个人的爱好,从小就喜欢玩游戏,更想自己去创作游戏。第一期只是介绍了一些基础,下一步就会来编写一个小游戏啦。
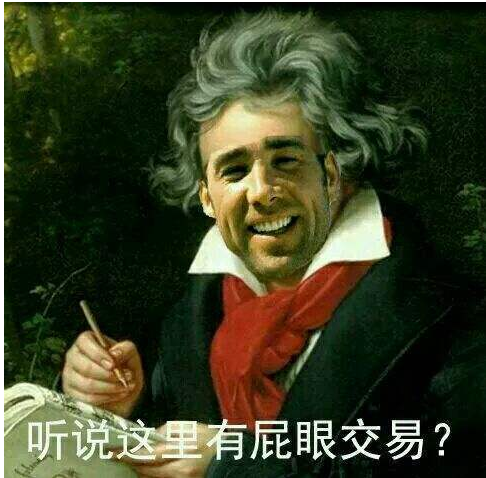