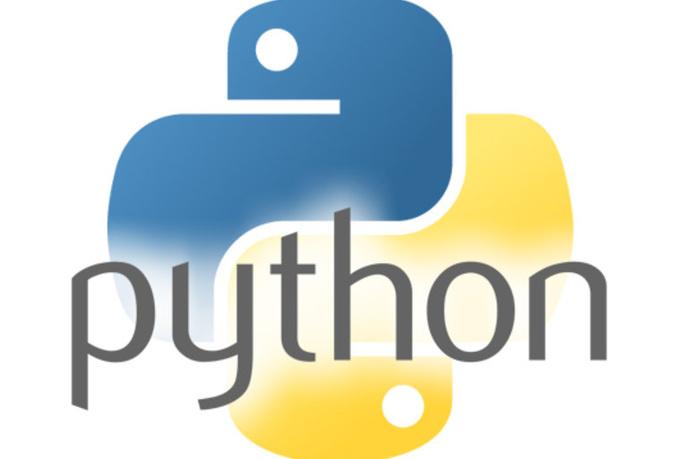
CSV文件
CSV (Comma‐Separated Value, 逗号分隔值)
CSV是一种常见的文件格式,用来存储批量数据
1. 文件保存
np.savetxt(frame, array, fmt='%.18e', delimiter=None)
• frame : 文件、字符串或产生器,可以是.gz或.bz2的压缩文件
• array : 存入文件的数组
• fmt : 写入文件的格式,例如:%d %.2f %.18e
• delimiter : 分割字符串,默认是任何空格
栗子
a=np.arange(100).reshape(5,20)
np.savetxt('a.csv',a,fmt='%d',delimiter=',')
生成文件
0,1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19
20,21,22,23,24,25,26,27,28,29,30,31,32,33,34,35,36,37,38,39
40,41,42,43,44,45,46,47,48,49,50,51,52,53,54,55,56,57,58,59
60,61,62,63,64,65,66,67,68,69,70,71,72,73,74,75,76,77,78,79
80,81,82,83,84,85,86,87,88,89,90,91,92,93,94,95,96,97,98,99
b=np.arange(100).reshape(5,20)
np.savetxt('b.csv',a,fmt='%.1f',delimiter=',')
#浮点型
0.0,1.0,2.0,3.0,4.0,5.0,6.0,7.0,8.0,9.0,10.0,11.0,12.0,13.0,14.0,15.0,16.0,17.0,18.0,19.0
20.0,21.0,22.0,23.0,24.0,25.0,26.0,27.0,28.0,29.0,30.0,31.0,32.0,33.0,34.0,35.0,36.0,37.0,38.0,39.0
40.0,41.0,42.0,43.0,44.0,45.0,46.0,47.0,48.0,49.0,50.0,51.0,52.0,53.0,54.0,55.0,56.0,57.0,58.0,59.0
60.0,61.0,62.0,63.0,64.0,65.0,66.0,67.0,68.0,69.0,70.0,71.0,72.0,73.0,74.0,75.0,76.0,77.0,78.0,79.0
80.0,81.0,82.0,83.0,84.0,85.0,86.0,87.0,88.0,89.0,90.0,91.0,92.0,93.0,94.0,95.0,96.0,97.0,98.0,99.0
2. 读取文件
np.loadtxt(frame, dtype=np.float, delimiter=None, unpack=False)
• frame : 文件、字符串或产生器,可以是.gz或.bz2的压缩文件
• dtype : 数据类型,可选
• delimiter : 分割字符串,默认是任何空格
• unpack : 如果True,读入属性将分别写入不同变量
c=np.loadtxt('b.csv',delimiter=',')
c
Out[55]:
array([[ 0., 1., 2., ..., 17., 18., 19.],
[ 20., 21., 22., ..., 37., 38., 39.],
[ 40., 41., 42., ..., 57., 58., 59.],
[ 60., 61., 62., ..., 77., 78., 79.],
[ 80., 81., 82., ..., 97., 98., 99.]])
c=np.loadtxt('b.csv',dtype=np.int,delimiter=',')
c
Out[57]:
array([[ 0, 1, 2, ..., 17, 18, 19],
[20, 21, 22, ..., 37, 38, 39],
[40, 41, 42, ..., 57, 58, 59],
[60, 61, 62, ..., 77, 78, 79],
[80, 81, 82, ..., 97, 98, 99]])
CSV只能有效存储一维和二维数组
np.savetxt() np.loadtxt()只能有效存取一维和二维数组
任意维度文件的存取
a.tofile(frame, sep='', format='%s')
• frame : 文件、字符串
• sep : 数据分割字符串,如果是空串,写入文件为二进制
• format : 写入数据的格式
a=np.arange(100).reshape(5,10,2)
a.tofile('b.dat',sep=',',format='%d')
0,1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20,21,22,23,24,25,26,27,28,29,30,31,32,33,34,35,36,37,38,39,40,41,42,43,44,45,46,47,48,49,50,51,52,53,54,55,56,57,58,59,60,61,62,63,64,65,66,67,68,69,70,71,72,73,74,75,76,77,78,79,80,81,82,83,84,85,86,87,88,89,90,91,92,93,94,95,96,97,98,99
a=np.arange(100).reshape(5,10,2)
a.tofile('b.dat',format='%d')
! " # $ % & ' ( ) * + , - . / 0 1 2 3 4 5 6 7 8 9 : ; < = > ? @ A B C D E F G H I J K L M N O P Q R S T U V W X Y Z [ \ ] ^ _ ` a b c
np.fromfile(frame, dtype=float, count=‐1, sep='')
• frame : 文件、字符串
• dtype : 读取的数据类型
• count : 读入元素个数,‐1表示读入整个文件
• sep : 数据分割字符串,如果是空串,写入文件为二进制
a=np.arange(100).reshape(5,10,2)
a.tofile('b.dat',sep=',',format='%d')
c=np.fromfile('b.dat',dtype=np.int,sep=',')
c
Out[70]: array([ 0, 1, 2, ..., 97, 98, 99])
c=np.fromfile('b.dat',dtype=np.int,sep=',').reshape(5,10,2)
c
Out[72]:
array([[[ 0, 1],
[ 2, 3],
[ 4, 5],
...,
[14, 15],
[16, 17],
[18, 19]],
...,
...,
[94, 95],
[96, 97],
[98, 99]]])
a=np.arange(100).reshape(5,10,2)
a.tofile('b.dat',format='%d')
c=np.fromfile('b.dat',dtype=np.int).reshape(5,10,2)
c
Out[76]:
array([[[ 0, 1],
[ 2, 3],
[ 4, 5],
...,
[14, 15],
[16, 17],
[18, 19]],
...,
...,
[94, 95],
[96, 97],
[98, 99]]])
该方法需要读取时知道存入文件时数组的维度和元素类型
a.tofile()和np.fromfile()需要配合使用
可以通过元数据文件来存储额外信息
numpy 便捷文件存取
np.save(fname, array) 或np.savez(fname, array)
• fname : 文件名,以.npy为扩展名,压缩扩展名为.npz
• array : 数组变量
np.load(fname)
• fname : 文件名,以.npy为扩展名,压缩扩展名为.npz
a=np.arange(100).reshape(5,10,2)
np.save('a.npy',a)
b=np.load('a.npy')
b
Out[80]:
array([[[ 0, 1],
[ 2, 3],
[ 4, 5],
...,
[14, 15],
[16, 17],
[18, 19]],